I’ve started to plunder my stash of Sega CD games for my Gaming Pathology project. To run the games with the Gens emulator under Windows it is necessary to either install ASPI drivers for accessing the game CD-ROMs in a particular manner, or rip the data and audio tracks into a particular filename sequence in order to play them directly from the hard disk. Since I couldn’t make the former work on my new machine, I proceeded with the latter option.
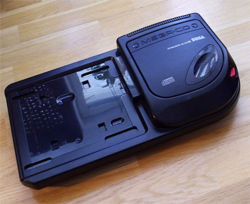
Gens wants the data track, i.e. ISO-9660 CD-ROM filesystem, as ‘title.iso’. Any redbook CD audio tracks after the data track need to be in the same directory, compressed as MP3, and named as ‘title 02.mp3’…’title nn.mp3’. After performing the process more or less manually for Revengers of Vengeance (I automated some parts, but had to manually rename the files in the end, and RoV has 44 audio tracks), I wrote a Python script to help me with other games (and I’m not very good at Python yet but I like these opportunities to learn).
There might be other ways, better ways, but this is my new way. The script relies on cdparanoia and LAME (oh, and dd and rm). I didn’t know any program to query a CD to learn how many audio tracks it had (except my own hacked up program and I didn’t feel like leveraging it), so I just perform a rip loop until cdparanoia returns an error. LAME is instructed to encode at its ‘insane’ profile, sparing no bitrate. Syntax is ‘./rip-sega-cd.py “game title”‘ which will produce an ISO file and a series of MP3 files if redbook audio is present:
$ ./rip-sega-cd.py "Revengers Of Vengeance" ripping Revengers Of Vengeance ripping data track... /bin/dd if=/dev/cdrom of="Revengers Of Vengeance.iso" ripping audio tracks... /usr/bin/cdparanoia --quiet 2 /usr/bin/lame --quiet --preset insane cdda.wav "Revengers Of Vengeance 02.mp3" /bin/rm cdda.wav [...repeated for each redbook CD audio track...]