Last year, I delved into code coverage tools and their usage with FFmpeg. I learned about using GNU gcov, which is powerful but pretty raw about the details it provides to you. I wrote a script to help interpret its output and later found another script called gcovr to do the same, only much better.
I later found another tool called lcov which is absolutely amazing for understanding code coverage of your software. I’ve been meaning to use it to further FATE test coverage for the multimedia projects.
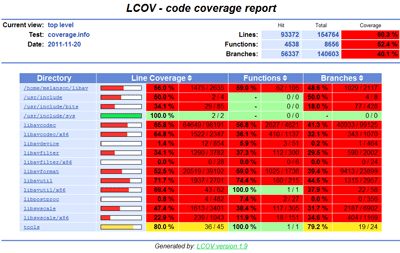
Click for larger image
Basic Instructions
Install the lcov tool, of course. In Ubuntu, 'apt-get install lcov'
will do the trick.
Build the project with code coverage support, i.e.,
./configure --enable-gpl --samples=/path/to/fate/samples \ --extra-cflags="-fprofile-arcs -ftest-coverage" \ --extra-ldflags="-fprofile-arcs -ftest-coverage" make
Clear the coverage data:
lcov --directory . --zerocounters
Run the software (in this case, the FATE test suite):
make fate
Let lcov work its magic:
lcov --directory . --capture --output-file coverage.info mkdir html-output genhtml -o html-output coverage.info
At this point, you can aim your web browser at html-output/index.html to learn everything you could possibly want to know about code coverage of the test suite. You can sort various columns in order to see which modules have the least code coverage. You can drill into individual source files and see highlighted markup demonstrating which lines have been executed.
As you can see from the screenshot above, FFmpeg / Libav are not anywhere close to full coverage. But lcov provides an exquisite roadmap.