It’s the Thanksgiving holiday here in the United States. I guess that’s as good a reason as any to release a first cut of my VP8 encoder. In order to remind people that they shouldn’t expect phenomenal quality from it — and to discourage inexperienced people from trying to create useful videos with it — I have hardcoded the quantizers to their maximum settings. For those not skilled in the art, this is the setting that yields maximum compression and worst quality. When compressing the Big Buck Bunny logo image, the resulting file is only 2839 bytes but observe the reconstructed quality:
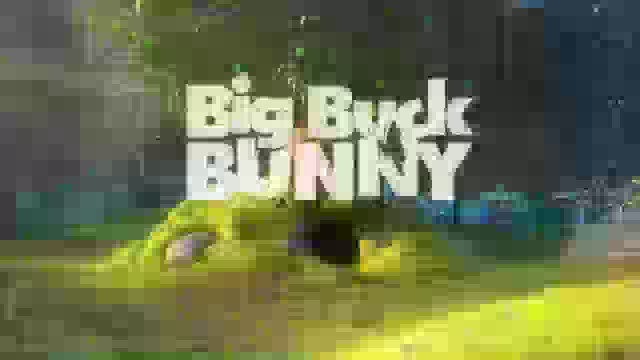
It really just looks like a particularly stormy day in the forest.
First VP8 File From An Independent Encoder
I found a happy medium on the quantizer scale and encoded the first 30 seconds of Big Buck Bunny for your inspection. I guess this makes it the first VP8/WebM file from an independent encoder (using FFmpeg’s Matroska muxer as well).
Download: bbb-360p-30sec-q40.webm (~13 MBytes)
I think the quality makes it look like it was digitized from an old VHS tape.
For fun, here’s the version with the quantizer cranked to the max: bbb-360p-30sec-q127.webm (~1.3 MBytes)
Aside: I was going to encapsulate the video in this post using a bare HTML5 <video> tag for the benefit of the small browsing population who could view that (indeed, it works fine in Chrome). But that would be insane due to the fact that supporting browsers preload the video with no easy (read: without the help of JavaScript) method for overriding this unacceptable default.
The Code
I’m still trying to get over my fear of git. To that end, I have posted the code on Github:
https://github.com/multimediamike/ffvp8enc
I still don’t like you, git. But I’m sure we’ll find some way to make this work.
Other required code changes in the basic FFmpeg tree:
- Of course, copy vp8enc.c into libavcodec/
- In libavcodec/allcodecs.c, ‘
REGISTER_DECODER (VP8, vp8);
‘ turns into ‘REGISTER_ENCDEC (VP8, vp8);
‘ - Add ‘
OBJS-$(CONFIG_VP8_ENCODER) += vp8enc.o
‘ to libavcodec/Makefile
Further Work
About the limitations and work yet to do:
- it’s still intra-only, no interframes (which is where a lot of compression occurs)
- no rate control or distortion optimization, obviously
- no intra 4×4 coding (that’s close to working but didn’t my little T-day deadline)
- no quantization control; this should really be hooked up to the FFmpeg command line but I’m not sure how
- encoder writes into a static-sized, 1/2 MB memory buffer; this can overflow
- code is a mess (what did you expect at this stage of the game?)
- lots and lots of other things, surely